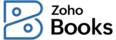
Software Development Kit methods
IN THIS PAGE…
Init
This is the first method to add for initializing the widgets.
var ZbooksClient = ZFAPPS.extension.init();
ZbooksClient.then(function(app) {
/* Below is an example to get the user name */
ZFAPPS.get('organization').then(function (data) {
//response Handling
}).catch(function (err) {
//error Handling
});
});
Get
This method is used to request data from the source. In Zoho Books, you can fetch the details of the organization, user, invoice and other entities using this method. You can look into the different ‘get’ supported entity keys here.
ZFAPPS.get('invoice').then(function (data) {
//response Handling
}).catch(function (err) {
//error Handling
});
Response:
{
"invoice": {
"invoice_id": "112233",
"invoice_number": "INV-000006",
"salesorder_id": "",
"salesorder_number": "",
"crm_owner_id": "",
"zcrm_potential_id": "",
"zcrm_potential_name": "",
"date": "2018-08-25",
"date_formatted": "25 Aug 2019",
"status": "paid",
"status_formatted": "Paid",
"amount": 101,
"amount_formatted": "$101.00",
.......
}
}
Set
The set method is used to set a module param while creating the transaction. For example, In Zoho Books, while creating the invoice you can modify the invoice params using this set handler.
To set invoice reference number from rendered sidebar widget:
ZFAPPS.set('invoice.reference_number', '001122').then(function (data) {
//response Handling
}).catch(function (err) {
//error Handling
});
Response:
{
"invoice": {
"invoice_id": "112233",
"invoice_number": "INV-00006",
"reference_number": "001122",
.......
}
Request
This method is used to invoke a third-party API or Zoho Books API from the widget. The connection_link_name defined in the plugin-manifest.json file will be invoked.
Notes: Sometimes, you need data from a third-party service that doesn’t need authorization. In that case, you do not need to add connection_link_name in the options.
var options = {
url: 'https://desk.zoho.com/api/v1/tickets/',
method: "GET/PUT/POST/DELETE" ,
url_query: [{
key: 'QUERY_NAME',
value: 'QUERY_VALUE'
}],
header:[{
key: 'header-key',
value: 'header-value'
}],
body: {
mode: 'urlencoded/formdata/raw',
urlencoded: [{
key: 'KEYNAME',
value: 'VALUE',
type: 'encodedType'
}],
formdata: [{
key: 'KEYNAME',
value: 'VALUE'
}],
raw: 'RAWDATA'
},
connection_link_name: 'zohodeskconnection'
};
ZFAPPS.request(options).then(function(value) {
//response Handling
let responseJSON = JSON.parse(value.data.body);
}).catch(function(err) {
//error Handling
});
Response:
{
"code": "",
"message": "Triggered Successfully",
"data":{
"body": {} // response of third party service
}
}
Name | Type | Description | ||||||||||||||||||||||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
options | Object |
|
Invoke
The invoke method is used to perform custom actions. It accepts two arguments, the name of an event that has to be trigged as the first argument and the corresponding value to the event that is passed as an object for the second argument.
Currently Zoho Books supports RESIZE and REFRESH_DATA options.
Resize
By default, Zoho Books supports a sidebar widget with a width of 350px. In case, you want to resize the widget, you can invoke the below RESIZE method:
ZFAPPS.invoke('RESIZE', { width: '100px', height: '100px'})
.then(() => { console.log('Resized successfully');
});
Refresh Data
Let’s say you have a widget in the invoice details sidebar, and you want to trigger an API for changing the status of an invoice from Draft to Sent, but the status has not been updated in the invoice details page. In that case, to view the updated changes, the page needs to be reloaded.
Here is an example for refreshing the invoice details page:
ZFAPPS.invoke('REFRESH_DATA', 'invoice')
.then(() => { console.log('Reloaded successfully');
});
Data Storage
Sometimes, the widgets you create might require data storage and retrieval capabilities. To help you in such cases, a data store is available to set store and get retrieve data.
The following APIs provide database management functionalities to widgets:
Notes: The key’s value can be a maximum of 20 characters.
Store
ZFAPPS.store(`api_key`, {
value: 'xxxxx'
}).then(function (data) {
//response Handling
}).catch(function (err) {
//error Handling
});
ZbooksClient.then(async function(App) {
let user = await ZFAPPS.get('user.zuId');
let user_id = user['user.zuId'];
ZFAPPS.store(`api_key_${user_id}`, {
value: 'xxxxx',
visiblity: 'onlyme'
}).then(function (data) {
//response Handling
}).catch(function (err) {
//error Handling
});
});
Response:
{
"messgage": "Stored Successfully",
"api_key_XXXXX": "xxxxx"
}
Argument Name | Data Type | Description | ||||||
---|---|---|---|---|---|---|---|---|
Argument 1(Key) | String | Data to be stored corresponding to the key. | ||||||
Argument 2 (ValueObject) |
Object
|
Retrieve
Retrieves data from the data store.
ZFAPPS.retrieve('api_key').then(function (data) {
//response Handling
}).catch(function (err) {
//error Handling
});
//If visibllity of param is onlyme, append the user_id to retrive it
ZbooksClient.then(async function(App) {
let user = await ZFAPPS.get('user.zuId');
let user_id = user['user.zuId'];
ZFAPPS.retrieve(`api_key_${user_id}`).then(function (data) {
//response Handling
}).catch(function (err) {
//error Handling
});
});
Response:
{
"messgage": "Retrived Successfully",
"api_key_XXXXX": "xxxxx"
}
Event APIs
You can configure the widget to receive information when an event occurs, such as, when adding a line item in an invoice or changing the customer while creating the invoice.
On Change
While you add/modify the input params like customer, entity_number, date, items etc., in Zoho Books, it’ll broadcast the event change. You can see the event and process the updated data.
Supported modules:
Invoice - ON_INVOICE_CHANGE
Estimate - ON_ESTIMATE_CHANGE
Sales orders - ON_SALESORDER_CHANGE
Customer - ON_CUSTOMER_CHANGE
Vendor - ON_VENDOR_CHANGE
Creditnote - ON_CREDITNOTE_CHANGE
Retainer Invoice - ON_RETAINERINVOICE_CHANGE
ZbooksClient.then(function(App) {
App.instance.on('ON_INVOICE_CHANGE', function () {
// Actions
});
});
On Preview
In the transaction details page, when the customer transitions between the entity records, Zoho Books will broadcast the on_preview event. So you can see the event and process the updated data.
Supported modules:
Invoice - ON_INVOICE_PREVIEW
Estimate - ON_ESTIMATE_PREVIEW
Salesorder - ON_SALESORDER_PREVIEW
Customer - ON_CUSTOMER_PREVIEW
Vendor - ON_VENDOR_PREVIEW
Creditnote - ON_CREDITNOTE_PREVIEW
Retainer Invoice - ON_RETAINERINVOICE_PREVIEW
ZbooksClient.then(function(App) {
App.instance.on('ON_INVOICE_PREVIEW', function () {
// Actions
});
});
Saved
After the transaction is created, Zoho Books will broadcast the saved event. You can watch the event and write the required action to be triggered after the transaction is saved.
Notes: The saved event can be seen only by the background widgets.
Supported modules:
Invoices - INVOICE_SAVED
Estimates - ESTIMATE_SAVED
Salesorder - SALESORDER_SAVED
Customer - CUSTOMER_SAVED
Vendor - VENDOR_SAVED
Creditnote - CREDITNOTE_SAVED
Retainer Invoice - RETAINERINVOICE_SAVED
ZbooksClient.then(function(App) {
App.instance.on('INVOICE_SAVED', function () {
// Actions
});
});
Pre Save
After the transaction is created, Zoho Books will broadcast before the saved event. You can watch the event and write the required action to be triggered before the transaction is saved.
Notes: The saved event can be seen only by the background widgets.
Supported modules:
Invoices - ON_INVOICE_PRE_SAVE
ZbooksClient.then(function(App) {
App.instance.on('ON_INVOICE_PRE_SAVE', function () {
// Actions Return false if you want to prevent from saving
return new Promise((resolve, reject) => {
resolve({
prevent_save: false,
});
});
});
});
Modal
Show Modal
Display widget as a modal in the application.
Notes: If you want to display the widget as a modal instead of a sidebar, you’ll have to add “widget_type” : “modal” in the plugin-manifest.json for that specific widget.
ZbooksClient.then(function(App) {
ZFAPPS.showModal({
url: "/app/modal.html#/?route=modal"
});
});
Close Modal
You can use the following method to close the modal.
ZbooksClient.then(function(App) {
ZFAPPS.closeModal();
});